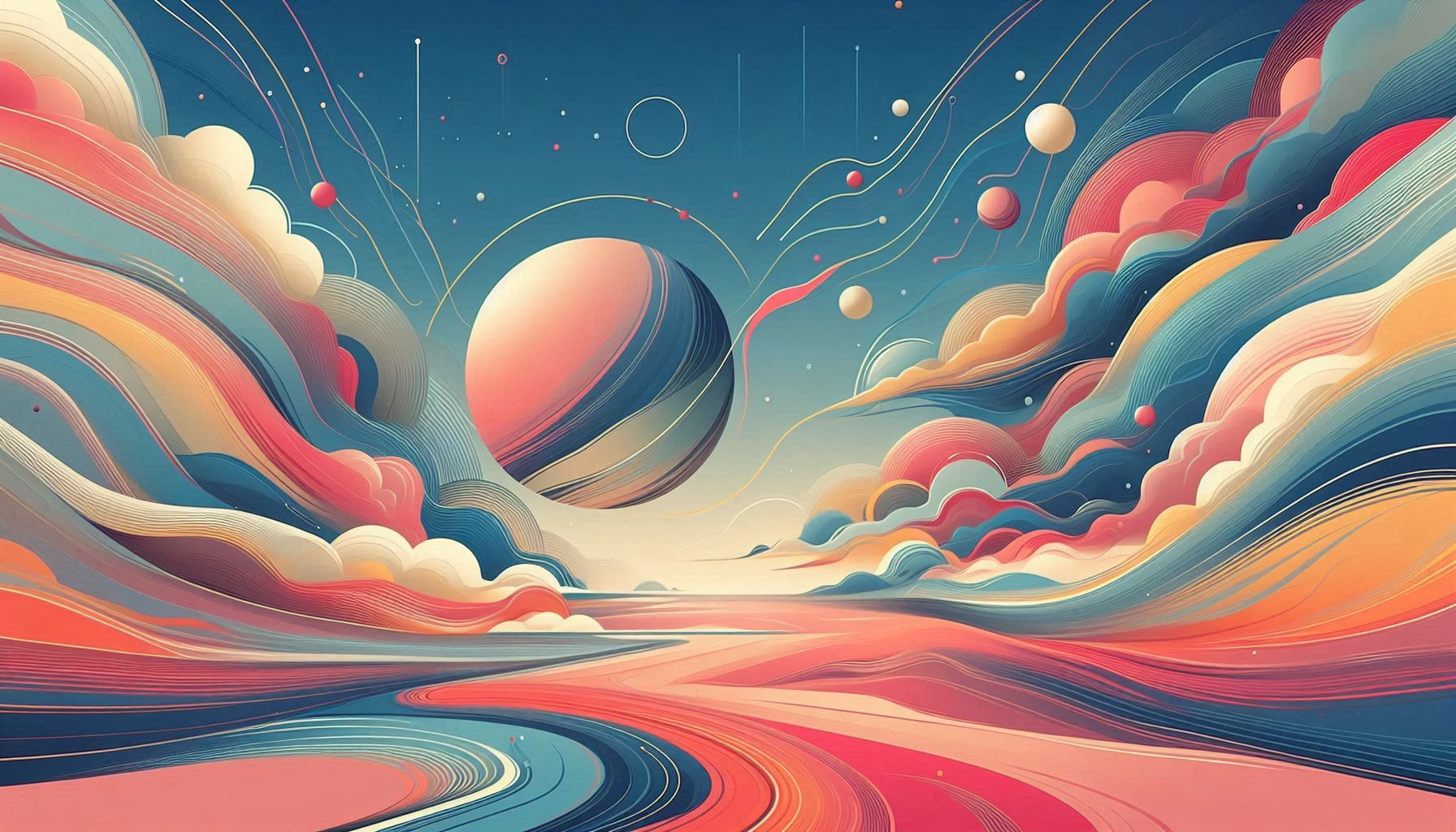
Setting up Circuit Playground Express
Introduction
Circuit Playground Express is one of the best beginner boards available today. Small form factor that contains a variety of sensors and on top of it the ability to use various dev tools to build.
This post is about setting up your new board and start developing using CircuitPython
Sanity Check
Once getting the board out of the box, connect it to a laptop with USB. Assuming you don’t have any relevant software installed, the device wouldn’t come up in your explorer.
At this moment the factory installed boot loader will execute and you will see LEDs flashing in a synchronous fashion. Try pushing buttons around except the small reset button.
Arduino
Before we jump into circuit python it is better to have a low level development system in place as well to cover all the basic drivers needed for the board. To do that we will use Arduino, download the latest version for your OS and install.
Once installed, launch the IDE while the board is still connected via USB. The Arduino board manager should kick in and auto-detect the board. This will prompt the installation of relevant packages.
After the installation, quit the Arduino IDE.
Mu Editor
For beginners, it is better to stick with default recommendations. Mu Editor is the recommended code editor for circuit python so I am gonna go with it. Download, install and run as usual. On launch select circuit python from the mode section. (PS: the first launch will take some time).
Also, make sure that python is installed and set up on the machine before installing the mu editor.
Reset !
Once everything is installed and ready to use, press the reset button on your board. The small button in between the two big ones and just beside the MCU labelled reset. First press it once and if nothing happens then try double tapping. Resetting the board will stop the LEDs from flashing and the device will appear in explorer.
You can now open the device and see three files stored in it. Copy and take a backup of files including the CURRENT.UF2
which is kind of a boot loader for the board.
Read this to know more about UF2
CircuitPython UF2
Installing CircuitPython to the board is simply replacing the CURRENT.UF2
with the circuit python one for your board. Download it from the circuitpython.org
It is basically a python interpreter along with some basic libraries that lets you run Python code directly on the board and program it using high level libraries.
To install, drop the UF2
file into the device and wait for the device name to change.
Editing Code
Once loaded you could see the code.py
file in the device ready for editing. Open the Mu Editor again and it will recognise that a compatible board is connected. Load
the code.py file from the device location and add your own logic.
Refer to the very basic LED blink example given here.
# https://learn.adafruit.com/welcome-to-circuitpython/creating-and-editing-code
import board
import digitalio
import time
led = digitalio.DigitalInOut(board.LED)
led.direction = digitalio.Direction.OUTPUT
while True:
led.value = True
time.sleep(0.5)
led.value = False
time.sleep(0.5)
As soon as you save the code the LED should start blinking. That’s it, you are done.